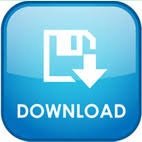
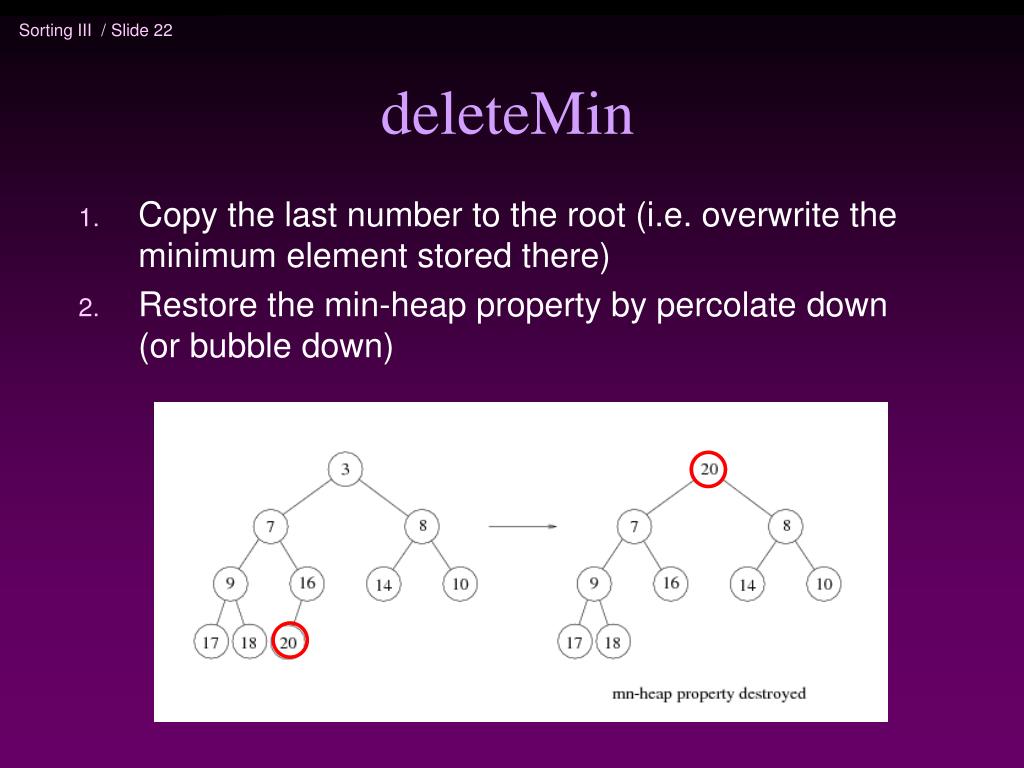
Next, we check whether each of the passed in strings are the word “sir”.
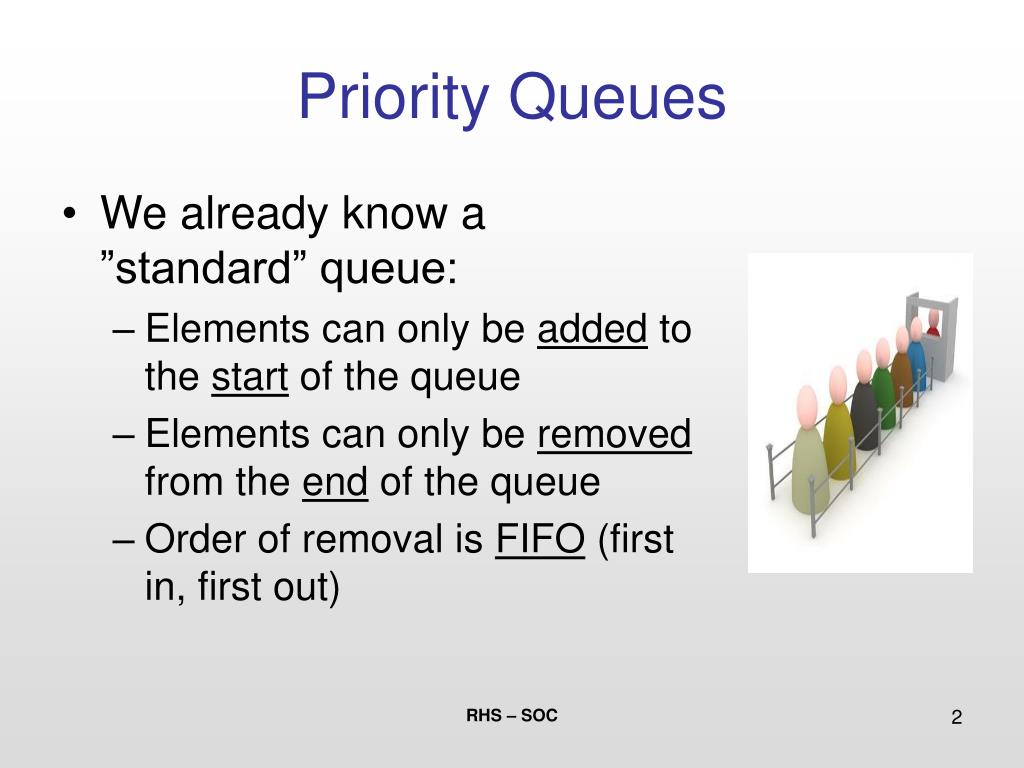
We simply inherit from IComparer, where T is the type we are comparing. If (titleAIsFancy = titleBIsFancy) //If both are fancy (Or both are not fancy, return 0 as they are equal)Įlse if (titleAIsFancy) //Otherwise if A is fancy (And therefore B is not), then return -1Įlse //Otherwise it must be that B is fancy (And A is not), so return 1 Var titleBIsFancy = titleB.Equals("sir", StringComparison.InvariantCultureIgnoreCase)
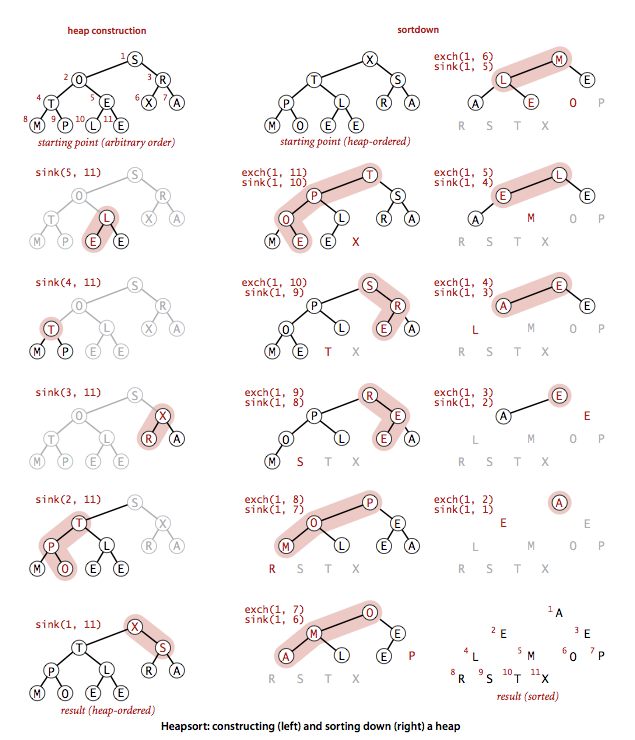
Var titleAIsFancy = titleA.Equals("sir", StringComparison.InvariantCultureIgnoreCase) Public int Compare(string titleA, string titleB)
Priority queue time complexity code#
For that, this piece of code should do the trick : class TitleComparer : IComparer The first thing we need to do is work out a way to compare titles. Even if they show up at the back of the queue, they should get served first (Disgusting I know!). This is a fancy bank in the middle of London city, and therefore there is priority serving of anyone with the title of “Sir” in their name. If a higher-priority task is added, it should be the next task to run after the currently-running task completes, regardless of its position in the queue. Let’s assume that we are building a banking application. However, there is a complexity: each task has a priority, and higher-priority tasks should jump the queue. But what if we have complex logic on how priority should be derived? We could build this logic ourselves and still use an integer priority, or we could use a custom comparer. The above example is relatively easy to comprehend since the priority is nothing but an integer. It really is that simple! Using Custom Comparers It stores the elements in a sorted order where the first element of the queue is the greatest of all. I wish I could extend out this bit of the tutorial but. A priority queue is a type of dynamic container in STL. This procedure is often called heapify and works on an pre existing array that is used to construct a binary tree on it in O(n) time.The lower the integer, the higher the priority, and we can see our items are always popped based on this priority regardless of the order they were added to the queue. To the claim of O(n) time for construction is correct (as stated by in the comments). openjdk-mirror/jdk7u-jdk/blob/master/src/share/. To get a guaranteed O(n log n) time for adding n elements you may state the size of your n elements to omit extension of the container: PriorityQueue(int initialCapacity) from the source code of java PriorityQueue, its actually using SiftUp which be NLogN solution. I would assume that the growth will cost O(n) time, which then would also be the worst case time complexity for. The details of the growth policy are not specifiedĪs they state in the Doc as well, the PriorityQueue is based on an array with a specific initial capacity. You are right to question the bounds as the Java Doc also states to the extension of this unbound structure: These time complexities seem all worst case ( wiki), except for. Generally, The time complexity of operations like insertion and deletion in the. However, the highest element is always the default in the C++ STL. O(1) for the retrieval methods (peek, element, and size) Priority Queue is a standard template library (STL) container in C++, in which the top element is either the largest or the smallest of all the elements (depending on the type of the priority queue). Let’s say if X is a parent node of Y, then the value of X follows some specific order with respect to value of Y and the same order will be followed across the tree. Using unordered Array: In unordered array deletion takes O (n) time complexity because it search for the element in Queue for the deletion and enqueue takes o (1) time complexity. O(n) for the remove(Object) and contains(Object) methods Priority-queue Heaps: A heap is a specific tree based data structure in which all the nodes of tree are in a specific order. We can then know the time complexities are as follows: Heapify an unordered array: If the collection is passed to the constructor, the time complexity will be O ( n ). O(log n) time for the enqueing and dequeing methods (offer, poll, 1 Answer Sorted by: 7 The PriorityQueue class is implemented as a min heap: Implements an array-backed, quaternary min-heap. It seems like the insertion of n elements should be O(n log n)
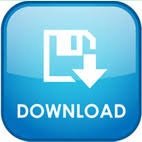